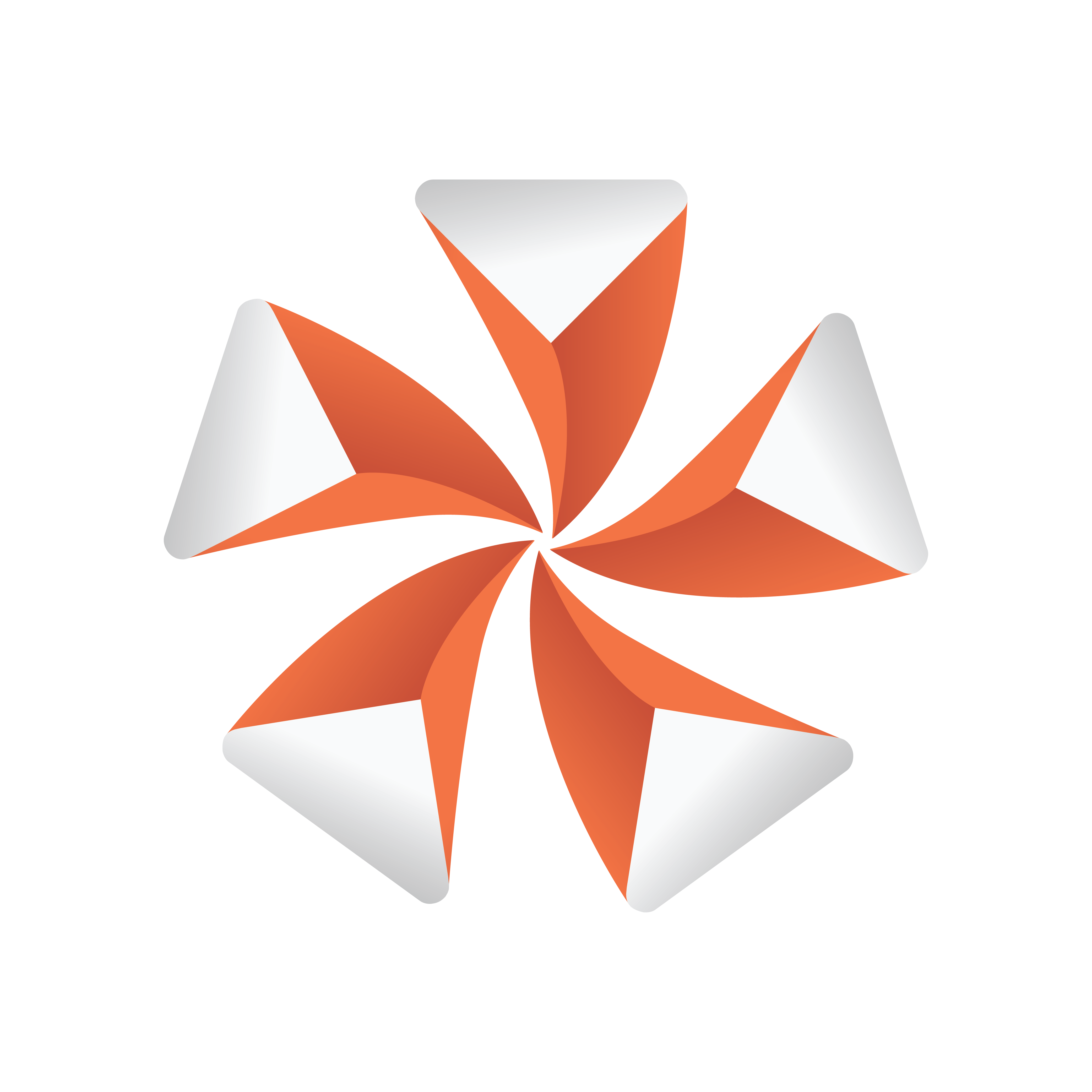
Viz Artist
Version 3.10 | Published May 03, 2018 ©
Data Sharing
This section describes the data sharing mechanism provided by Shared Memory Maps (SHM). A SHM is a map which holds user defined variables indexed by a string. It is exposed to the script interface via the Shared Memory data type. The variables are stored as Variant objects and thus can hold objects of any other type.
There are three types of SHM and the difference between them is their distribution locations:
-
Scene.Map: Referenced in plug-ins as Scene. This is the map local to the current Scene only. Every Scene has one map that can be used to exchange data among the scripts in the Scene.
-
System.Map: Referenced in plug-ins as Global. The system-wide map allows for data sharing among the scenes currently loaded into memory (e.g. data sharing between scenes in different layers).
-
VizCommunication.Map: Referenced in plug-ins as Distributed. A distributed map that enables data sharing among the Viz Engines connected to the same Graphic Hub. The Viz Engines must be logged in with users from the same Graphic Hub group.
This section contains information on the following topics:
VizCommunication.Map
The map exposed by the VizCommunication type is used to share data among the client computers connected to a Graphic Hub. Whenever a script on one client adds a new value or changes an existing value in the map, the change is propagated to the other clients via database messaging to update the local copy of each client’s map.
This does not necessarily mean that all local client maps are identical. When a client starts up after other clients have made changes to the VizCommunication.Map, it is not informed of those changes. See Synchronization in Shared Memory (SHM).
Map Access Through a TCP or UDP Interface
Elements in the distributed memory map (VizCommunication.Map) can be set (but not retrieved) through a TCP or UDP interface, exposed by Viz Artist. This option is particularly useful when writing external control applications.
For more information about map access through a TCP or UDP Interface, see External Data Input in Shared Memory (SHM).
Map Access through the Command Interface
There is limited support for getting and setting map elements through a command interface. The maps are accessible through these locations:
-
<scene>*MAP for the scene specific map
-
GLOBAL*MAP for system-wide map
-
VIZ_COMMUNICATION*MAP for the distributed map
A full list of supported commands is at: <viz install folder>\Documentation\CommandInterface\index.html
From the list of commands, the commands CLEAR, DELETE_ELEMENT and PURGE_ELEMENT will only work when sent through the command interface of Viz Artist.
The command CLEAR must be run on each Engine where the MAP is to be reset (VIZ_COMMUNICATION*MAP CLEAR).
Map Access from Within plug-ins
Functions for getting and setting map elements are provided by the plug-in interface. For more information, refer to the plug-in API documentation in your Viz installation folder.
Script Examples
This section contains two script examples for Shared Memory (SHM):
Example I
This is an example that shows how a Scene map can be used to pass data from one script to another (within the same Scene).
This script defines the OnEnterSubContainer, which is called whenever the mouse-cursor enters any of its sub-containers. In it, the name of the subcontainer is stored in the Scene map under the key subcontainer_name.
‘ Script
1
:
Sub OnEnterSubContainer(subContainer as Container)
Scene.Map[
"subcontainer_name"
] = subContainer.Name
End Sub
Example II
This example shows how external data can be sent to all Viz Engines connected to a Graphic Hub, and how they can utilize the same data. The example also shows how to group together related external data into an array inside the Viz Engine. This is not the only way of managing variables. They could just as well be sent individually, which will then not demand extra variable splitting in a script.
Vizrt do not recommend this as a method for the import of large amounts of data. For more information see External Data Input.
To create and set a value shared by all Viz Engines, that are connected to the same Graphic Hub, send the following command through the Viz Engine Console:
send VIZ_COMMUNICATION*MAP SET_STRING_ELEMENT
"my_variable"
1.2
;
10.8
;
20.3
;
15.9
CONSOLE: answer <>
send VIZ_COMMUNICATION*MAP GET_STRING_ELEMENT
"my_variable"
CONSOLE: answer <
1.2
;
10.8
;
20.3
;
15.9
>
This example shows a command sent to the Viz Engine, that assigns a value to a variable named my_variable. This image shows a script that uses this variable, and assigns one of its values to a text container named MyText:
From outside a Viz Engine the shared memory can be accessed by a connection to the Viz Engine listener port 6100. For more information on ways to access shared memory, see Map Access through the Command Interface.
Set and retrieve data from the SHM through scripting
To Set a Value
Through a Command Console:
send VIZ_COMMUNICATION*MAP SET_STRING_ELEMENT
"my_variable"
1.2
;
10.8
;
20.3
;
15.9
or through Script:
VizCommunication.map[
"my_variable"
] =
1.2
;
10.8
;
20.3
;
15.9
To Retrieve a Value
Dim MyVariable as string
MyVariable = (string)VizCommunication.map[
"my_variable"
]
Here, string is used to format the data from the SHM to a text value.
To Split the Values into an Array
You can then split the data inside MyVariable into a table called MyArray:
Dim MyArray as array [string]
MyVariable.split(
";"
,MyArray)
The information in MyVariable has now been split into four:
‘ MyArray[
0
] =
1.2
‘ MyArray[
1
] =
10.8
‘ MyArray[
2
] =
20.3
‘ MyArray[
3
] =
15.9
To Assign a Value to a Text Container
One of these values can then be assigned to a text container:
Scene.FindContainer(
"MyText"
).geometry.text = MyArray[
1
]
To Automatically Update Graphics
When a SHM variable is updated you will have to register the value for updates in the scene:
Scene.Map.RegisterChangedCallback(
"my_variable"
)
Changes to my_variable will execute a subroutine called OnSharedMemoryVariableChanged. This subroutine can do whatever you decide with the data. For further details, refer to the callback procedures described in the plug-in API documentation.
Receiving Notification on Map Changes
Sometimes you want to be notified when a value in an SHM changes, so you do not have to poll for changes within OnExecPerField. This is done by calling a map’s RegisterChangedCallback procedure, passing it the name of a key that you want to monitor. Now, whenever the variable identified by that key is changed, the OnSharedMemoryVariableChanged callback (if present) is invoked. Similarly, the OnSharedMemoryVariableDeleted callback is invoked, when the variable is deleted.
Example:
Sub OnInit()
Scene.Map.RegisterChangedCallback(
"subcontainer_name"
)
End Sub
Sub OnSharedMemoryVariableChanged(map as SharedMemory, mapKey as String)
If mapKey =
"subcontainer_name"
Then
Geometry.Text = (String)map[
"subcontainer_name"
]
End If
End Sub
OnSharedMemoryVariableChanged is passed to the map as well as the key whose variable has been changed.
Passing an empty string ("") to RegisterChangedCallback will make sure that OnSharedMemoryVariableChanged and OnSharedMemoryVariableDeleted is invoked whenever any variable in the map is modified or deleted:
Sub OnInit()
Scene.Map.RegisterChangedCallback(
""
)
End Sub
Sub OnSharedMemoryVariableChanged(map as SharedMemory, mapKey as String)
...
End Sub